PHP Tutorial
- PHP Tutorial
- Install PHP
- PHP Code
- PHP Echo and print
- PHP Variable
- PHP Variable Scope
- PHP $ and $$
- PHP Constants
- PHP Data Types
- PHP Operators
- PHP Comments
Control Statement
- PHP If else
- PHP Switch
- PHP For Loop
- PHP foreach loop
- PHP While Loop
- PHP Do While Loop
- PHP Break
- PHP Continue
PHP Functions
- PHP Functions
- Parameterized Function
- PHP Call By Value and reference
- PHP Default Arguments
- PHP Variable Arguments
- PHP Recursive Function
PHP Arrays
PHP Strings
PHP Math
PHP Form
PHP Include
State Management
PHP File
Upload Download
PHP OOPs Concepts
- OOPs Concepts
- OOPs Abstract Class
- OOPs Abstraction
- OOPs Access Specifiers
- OOPs Const Keyword
- OOPs Constructor and destructor
- Encapsulation
- Final Keyword
- OOPs Functions
- OOPs Inheritance
- OOPs Interface
- OOPs Overloading
- OOPs Type Hinting
PHP MySQLi
- MySQLi CONNECT
- MySQLi CREATE DB
- MySQLi CREATE Table
- MySQLi INSERT
- MySQLi UPDATE
- MySQLi DELETE
- MySQLi SELECT
- MySQLi Order by
PHP Topics
- Compound Types
- is_null() Function
- Special Types
- Inheritance Task
- Special Types
- MVC Architecture
- PHP vs. JavaScript
- PHP vs. HTML
- PHP vs. Node.js
- PHP vs Python
- PHP PDO
- Top 10 PHP frameworks
- phpMyAdmin
- Count All Array Elements
- Create Newline
- Get Current Page URL
PHP Mail
PHP do-while loop can be used to traverse set of code like php while loop. The PHP do-while loop is guaranteed to run at least once.
The PHP do-while loop is used to execute a set of code of the program several times. If you have to execute the loop at least once and the number of iterations is not even fixed, it is recommended to use the do-while loop.
It executes the code at least one time always because the condition is checked after executing the code.
The do-while loop is very much similar to the while loop except the condition check. The main difference between both loops is that while loop checks the condition at the beginning, whereas do-while loop checks the condition at the end of the loop.
Syntax
do{
//code to be executed
}while(condition);
Flowchart
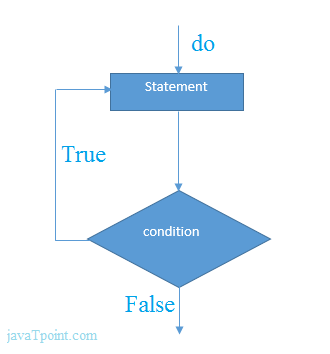
Example
<?php
$n=1;
do{
echo "$n<br/>";
$n++;
}while($n<=10);
?>
Output:
1 2 3 4 5 6 7 8 9 10
Example
A semicolon is used to terminate the do-while loop. If you don’t use a semicolon after the do-while loop, it is must that the program should not contain any other statements after the do-while loop. In this case, it will not generate any error.
<?php
$x = 5;
do {
echo "Welcome to protutorials! </br>";
$x++;
} while ($x < 10);
?>
Output:
Welcome to protutorials! Welcome to protutorials! Welcome to protutorials! Welcome to protutorials! Welcome to protutorials!
Example
The following example will increment the value of $x at least once. Because the given condition is false.
<?php
$x = 1;
do {
echo "1 is not greater than 10.";
echo "</br>";
$x++;
} while ($x > 10);
echo $x;
?>
Output:
1 is not greater than 10. 2
Difference between while and do-while loop
while Loop | do-while loop |
---|---|
The while loop is also named as entry control loop. | The do-while loop is also named as exit control loop. |
The body of the loop does not execute if the condition is false. | The body of the loop executes at least once, even if the condition is false. |
Condition checks first, and then block of statements executes. | Block of statements executes first and then condition checks. |
This loop does not use a semicolon to terminate the loop. | Do-while loop use semicolon to terminate the loop. |