PHP Tutorial
- PHP Tutorial
- Install PHP
- PHP Code
- PHP Echo and print
- PHP Variable
- PHP Variable Scope
- PHP $ and $$
- PHP Constants
- PHP Data Types
- PHP Operators
- PHP Comments
Control Statement
- PHP If else
- PHP Switch
- PHP For Loop
- PHP foreach loop
- PHP While Loop
- PHP Do While Loop
- PHP Break
- PHP Continue
PHP Functions
- PHP Functions
- Parameterized Function
- PHP Call By Value and reference
- PHP Default Arguments
- PHP Variable Arguments
- PHP Recursive Function
PHP Arrays
PHP Strings
PHP Math
PHP Form
PHP Include
State Management
PHP File
Upload Download
PHP OOPs Concepts
- OOPs Concepts
- OOPs Abstract Class
- OOPs Abstraction
- OOPs Access Specifiers
- OOPs Const Keyword
- OOPs Constructor and destructor
- Encapsulation
- Final Keyword
- OOPs Functions
- OOPs Inheritance
- OOPs Interface
- OOPs Overloading
- OOPs Type Hinting
PHP MySQLi
- MySQLi CONNECT
- MySQLi CREATE DB
- MySQLi CREATE Table
- MySQLi INSERT
- MySQLi UPDATE
- MySQLi DELETE
- MySQLi SELECT
- MySQLi Order by
PHP Topics
- Compound Types
- is_null() Function
- Special Types
- Inheritance Task
- Special Types
- MVC Architecture
- PHP vs. JavaScript
- PHP vs. HTML
- PHP vs. Node.js
- PHP vs Python
- PHP PDO
- Top 10 PHP frameworks
- phpMyAdmin
- Count All Array Elements
- Create Newline
- Get Current Page URL
PHP Mail
MVC is a software architectural pattern for implementing user interfaces on computers. It divides a given application into three interconnected parts. This is done to separate internal representations of information from the ways information is presented to, and accepted from the user.
- MVC stands for “Model view And Controller“.
- The main aim of MVC Architecture is to separate the Business logic & Application data from the USER interface.
- Different types of Architectures are available. These are 3-tier Architecture, N-tier Architecture, MVC Architecture, etc.
- The main advantage of Architecture is Reusability, Security and Increasing the performance of Application.
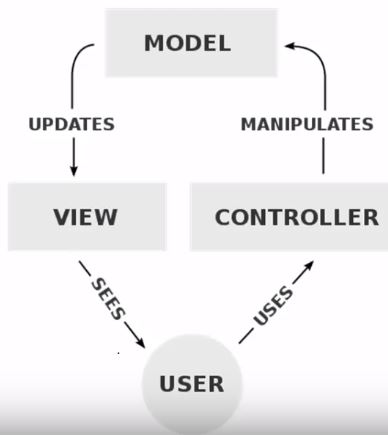
Model: Database operation such as fetch data or update data etc.
View: End-user GUI through which user can interact with system, i.e., HTML, CSS
Controller: Contain Business logic and provide a link between model and view.
Let’s understand this MVC concept in detail:
Model:
- The Model object knows all about all the data that need to be displayed.
- The Model represents the application data and business rules that govern to an update of data.
- Model is not aware about the presentation of data and How the data will be display to the browser.
View:
- The View represents the presentation of the application.
- View object refers to the model remains same if there are any modifications in the Business logic.
- In other words, we can say that it is the responsibility of view to maintain consistency in its presentation and the model changes.
Controller:
- Whenever the user sends a request for something, it always goes through Controller.
- A controller is responsible for intercepting the request from view and passes to the model for appropriate action.
- After the action has been taken on the data, the controller is responsible for directly passes the appropriate view to the user.
- In graphical user interfaces, controller and view work very closely together.