PHP Tutorial
- PHP Tutorial
- Install PHP
- PHP Code
- PHP Echo and print
- PHP Variable
- PHP Variable Scope
- PHP $ and $$
- PHP Constants
- PHP Data Types
- PHP Operators
- PHP Comments
Control Statement
- PHP If else
- PHP Switch
- PHP For Loop
- PHP foreach loop
- PHP While Loop
- PHP Do While Loop
- PHP Break
- PHP Continue
PHP Functions
- PHP Functions
- Parameterized Function
- PHP Call By Value and reference
- PHP Default Arguments
- PHP Variable Arguments
- PHP Recursive Function
PHP Arrays
PHP Strings
PHP Math
PHP Form
PHP Include
State Management
PHP File
Upload Download
PHP OOPs Concepts
- OOPs Concepts
- OOPs Abstract Class
- OOPs Abstraction
- OOPs Access Specifiers
- OOPs Const Keyword
- OOPs Constructor and destructor
- Encapsulation
- Final Keyword
- OOPs Functions
- OOPs Inheritance
- OOPs Interface
- OOPs Overloading
- OOPs Type Hinting
PHP MySQLi
- MySQLi CONNECT
- MySQLi CREATE DB
- MySQLi CREATE Table
- MySQLi INSERT
- MySQLi UPDATE
- MySQLi DELETE
- MySQLi SELECT
- MySQLi Order by
PHP Topics
- Compound Types
- is_null() Function
- Special Types
- Inheritance Task
- Special Types
- MVC Architecture
- PHP vs. JavaScript
- PHP vs. HTML
- PHP vs. Node.js
- PHP vs Python
- PHP PDO
- Top 10 PHP frameworks
- phpMyAdmin
- Count All Array Elements
- Create Newline
- Get Current Page URL
PHP Mail
There are 3 types of Access Specifiers available in PHP, Public, Private and Protected.
Public – class members with this access modifier will be publicly accessible from anywhere, even from outside the scope of the class.
Private – class members with this keyword will be accessed within the class itself. It protects members from outside class access with the reference of the class instance.
Protected – same as private, except by allowing subclasses to access protected superclass members.
EXAMPLE 1: Public
<?php
class demo
{
public $name="php";
functiondisp()
{
echo $this->name."<br/>";
}
}
class child extends demo
{
function show()
{
echo $this->name;
}
}
$obj= new child;
echo $obj->name."<br/>";
$obj->disp();
$obj->show();
?>
Output:
php
php
php
EXAMPLE 2: Private
<?php
classJavatpoint
{
private $name="Sonoo";
private function show()
{
echo "This is private method of parent class";
}
}
class child extends Javatpoint
{
function show1()
{
echo $this->name;
}
}
$obj= new child;
$obj->show();
$obj->show1();
?>
Output:
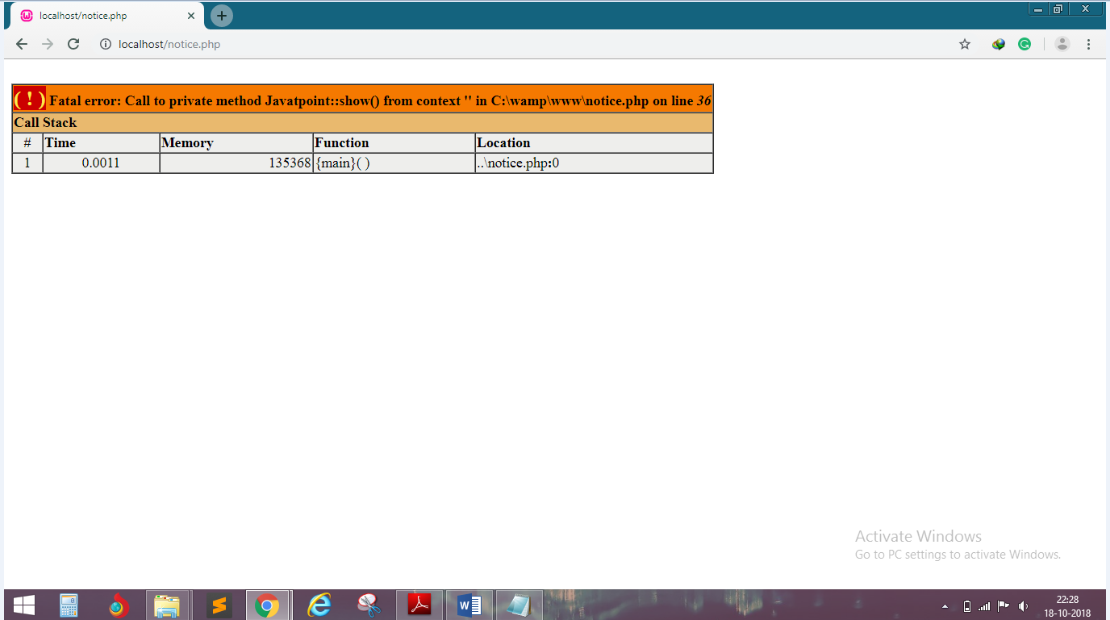
EXAMPLE 3: Protected
<?php
classJavatpoint
{
protected $x=500;
protected $y=100;
function add()
{
echo $sum=$this->x+$this->y."<br/>";
}
}
class child extends Javatpoint
{
function sub()
{
echo $sub=$this->x-$this->y."<br/>";
}
}
$obj= new child;
$obj->add();
$obj->sub();
?>
Output:
600
400
EXAMPLE 4: Public,Private and Protected
<?php
classJavatpoint
{
public $name="Ajeet";
protected $profile="HR";
private $salary=5000000;
public function show()
{
echo "Welcome : ".$this->name."<br/>";
echo "Profile : ".$this->profile."<br/>";
echo "Salary : ".$this->salary."<br/>";
}
}
classchilds extends Javatpoint
{
public function show1()
{
echo "Welcome : ".$this->name."<br/>";
echo "Profile : ".$this->profile."<br/>";
echo "Salary : ".$this->salary."<br/>";
}
}
$obj= new childs;
$obj->show1();
?>
Output:
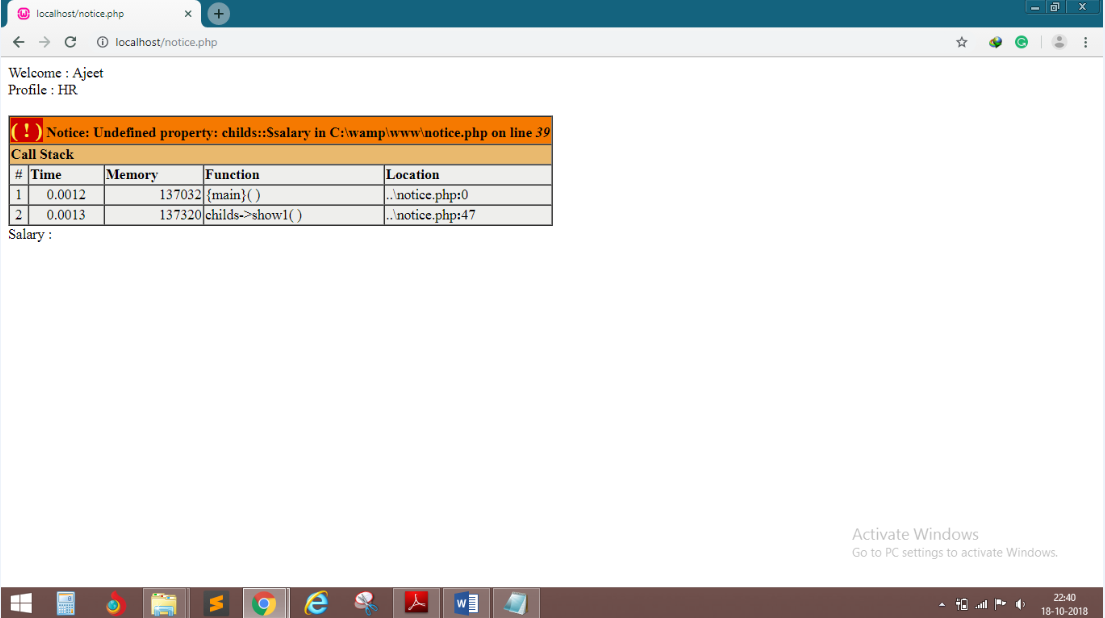