PHP Tutorial
- PHP Tutorial
- Install PHP
- PHP Code
- PHP Echo and print
- PHP Variable
- PHP Variable Scope
- PHP $ and $$
- PHP Constants
- PHP Data Types
- PHP Operators
- PHP Comments
Control Statement
- PHP If else
- PHP Switch
- PHP For Loop
- PHP foreach loop
- PHP While Loop
- PHP Do While Loop
- PHP Break
- PHP Continue
PHP Functions
- PHP Functions
- Parameterized Function
- PHP Call By Value and reference
- PHP Default Arguments
- PHP Variable Arguments
- PHP Recursive Function
PHP Arrays
PHP Strings
PHP Math
PHP Form
PHP Include
State Management
PHP File
Upload Download
PHP OOPs Concepts
- OOPs Concepts
- OOPs Abstract Class
- OOPs Abstraction
- OOPs Access Specifiers
- OOPs Const Keyword
- OOPs Constructor and destructor
- Encapsulation
- Final Keyword
- OOPs Functions
- OOPs Inheritance
- OOPs Interface
- OOPs Overloading
- OOPs Type Hinting
PHP MySQLi
- MySQLi CONNECT
- MySQLi CREATE DB
- MySQLi CREATE Table
- MySQLi INSERT
- MySQLi UPDATE
- MySQLi DELETE
- MySQLi SELECT
- MySQLi Order by
PHP Topics
- Compound Types
- is_null() Function
- Special Types
- Inheritance Task
- Special Types
- MVC Architecture
- PHP vs. JavaScript
- PHP vs. HTML
- PHP vs. Node.js
- PHP vs Python
- PHP PDO
- Top 10 PHP frameworks
- phpMyAdmin
- Count All Array Elements
- Create Newline
- Get Current Page URL
PHP Mail
Create program to find area of Triangle using inheritance.
Solution:
<?php
class getdata
{
public $h;
public $b;
public function method1()
{
$this->h= $_REQUEST['height'];
$this->b= $_REQUEST['base'];
}
}
classfindarea extends getdata
{
public $area;
public function method2()
{
$this->area= ($this->h* $this->b)/2;
echo "Area of Triangle is : ".$this->area;
}
}
if(isset($_REQUEST['submit']))
{
$obj = new findarea();
$obj->method1();
$obj->method2();
}
?>
<html>
<head>
</head>
<body>
<form method= "post">
<table align ="left" border="1">
<tr>
<td> Enter Height </td>
<td><input type = "text" name="height"/></td>
</tr>
<tr>
<td> Enter Base </td>
<td><input type = "text" name="base"/></td>
</tr>
<tr>
<td align ="center" colspan="2">
<input type= "submit"name="submit"value="click"/>
</td>
</tr>
</table>
</form>
</body>
</html>
Output:
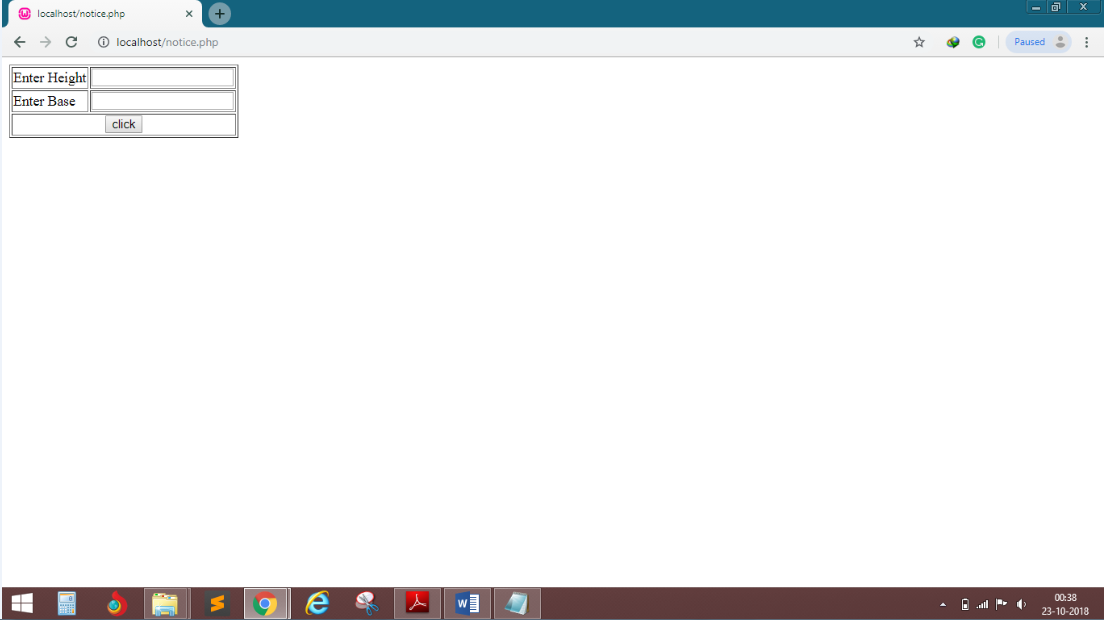
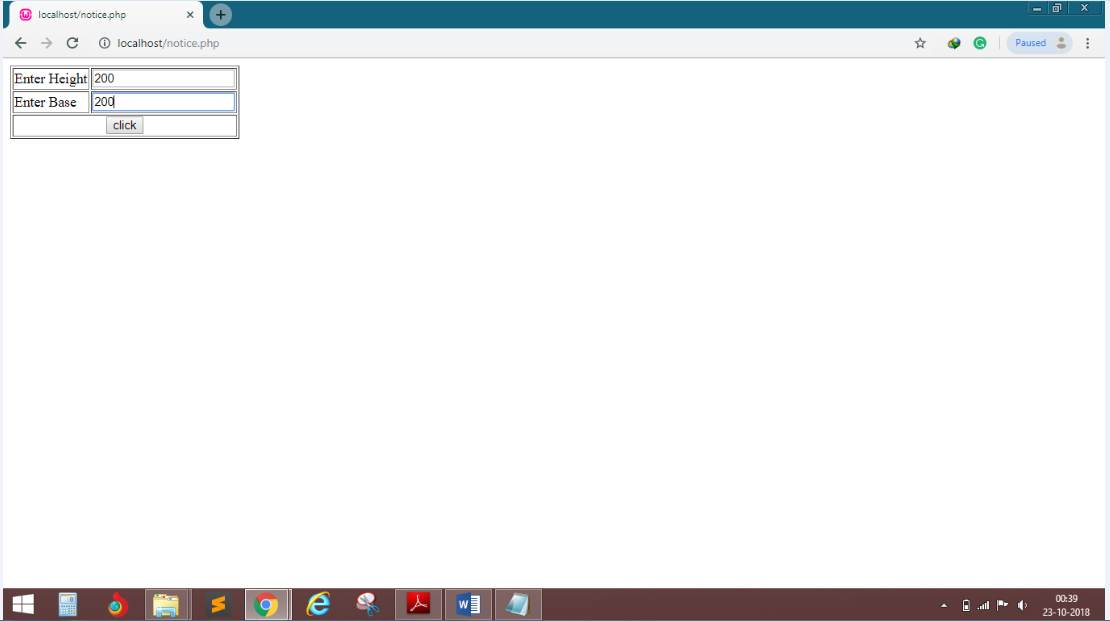
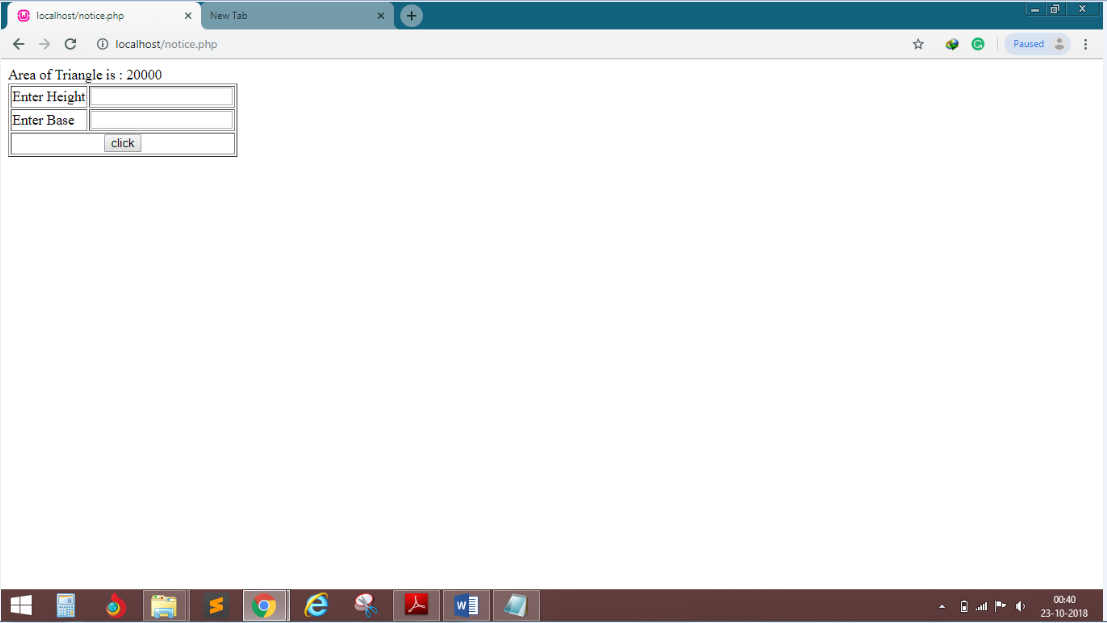