PHP Tutorial
- PHP Tutorial
- Install PHP
- PHP Code
- PHP Echo and print
- PHP Variable
- PHP Variable Scope
- PHP $ and $$
- PHP Constants
- PHP Data Types
- PHP Operators
- PHP Comments
Control Statement
- PHP If else
- PHP Switch
- PHP For Loop
- PHP foreach loop
- PHP While Loop
- PHP Do While Loop
- PHP Break
- PHP Continue
PHP Functions
- PHP Functions
- Parameterized Function
- PHP Call By Value and reference
- PHP Default Arguments
- PHP Variable Arguments
- PHP Recursive Function
PHP Arrays
PHP Strings
PHP Math
PHP Form
PHP Include
State Management
PHP File
Upload Download
PHP OOPs Concepts
- OOPs Concepts
- OOPs Abstract Class
- OOPs Abstraction
- OOPs Access Specifiers
- OOPs Const Keyword
- OOPs Constructor and destructor
- Encapsulation
- Final Keyword
- OOPs Functions
- OOPs Inheritance
- OOPs Interface
- OOPs Overloading
- OOPs Type Hinting
PHP MySQLi
- MySQLi CONNECT
- MySQLi CREATE DB
- MySQLi CREATE Table
- MySQLi INSERT
- MySQLi UPDATE
- MySQLi DELETE
- MySQLi SELECT
- MySQLi Order by
PHP Topics
- Compound Types
- is_null() Function
- Special Types
- Inheritance Task
- Special Types
- MVC Architecture
- PHP vs. JavaScript
- PHP vs. HTML
- PHP vs. Node.js
- PHP vs Python
- PHP PDO
- Top 10 PHP frameworks
- phpMyAdmin
- Count All Array Elements
- Create Newline
- Get Current Page URL
PHP Mail
PHP Parameterized functions are the functions with parameters. You can pass any number of parameters inside a function. These passed parameters act as variables inside your function.
They are specified inside the parentheses, after the function name.
The output depends upon the dynamic values passed as the parameters into the function.
PHP Parameterized Example 1
Addition and Subtraction
In this example, we have passed two parameters $x and $y inside two functions add() and sub().
<!DOCTYPE html>
<html>
<head>
<title>Parameter Addition and Subtraction Example</title>
</head>
<body>
<?php
//Adding two numbers
function add($x, $y) {
$sum = $x + $y;
echo "Sum of two numbers is = $sum <br><br>";
}
add(467, 943);
//Subtracting two numbers
function sub($x, $y) {
$diff = $x - $y;
echo "Difference between two numbers is = $diff";
}
sub(943, 467);
?>
</body>
</html>
Output:
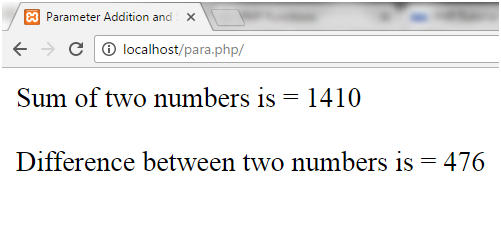
PHP Parameterized Example 2
Addition and Subtraction with Dynamic number
In this example, we have passed two parameters $x and $y inside two functions add() and sub().
Â
<?php
//add() function with two parameter
function add($x,$y)
{
$sum=$x+$y;
echo "Sum = $sum <br><br>";
}
//sub() function with two parameter
function sub($x,$y)
{
$sub=$x-$y;
echo "Diff = $sub <br><br>";
}
//call function, get two argument through input box and click on add or sub button
if(isset($_POST['add']))
{
//call add() function
add($_POST['first'],$_POST['second']);
}
if(isset($_POST['sub']))
{
//call add() function
sub($_POST['first'],$_POST['second']);
}
?>
<form method="post">
Enter first number: <input type="number" name="first"/><br><br>
Enter second number: <input type="number" name="second"/><br><br>
<input type="submit" name="add" value="ADDITION"/>
<input type="submit" name="sub" value="SUBTRACTION"/>
</form>
Output:
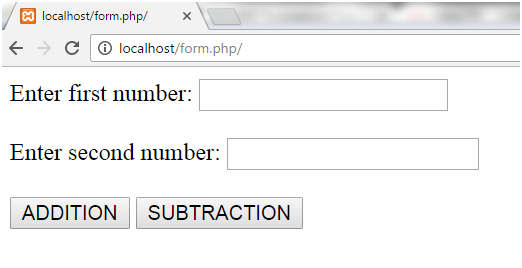
We passed the following number,
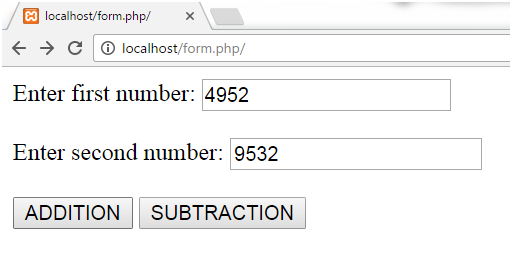
Now clicking on ADDITION button, we get the following output.
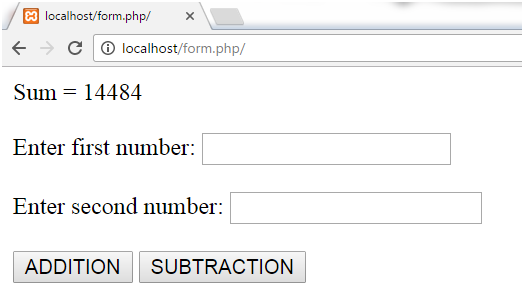
Now clicking on SUBTRACTION button, we get the following output.
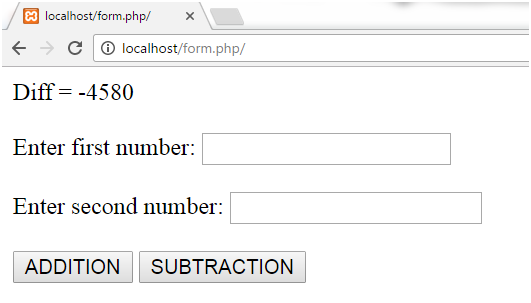